The Java KeyGenerator class (javax.crypto.KeyGenerator
) is used to generate symmetric encryption keys. A symmetric encryption key is a key that is used for both encryption and decryption of data, by a symmetric encryption algorithm. In this Java KeyGenerator tutorial I will show you how to generate symmetric encryption keys.
- Key Pair Generator Java Example
- Java Code Generator
- Random Word Generator Java
- Java Random Number Generator Code
Creating a KeyGenerator Instance
Before you can use the Java KeyGenerator
class you must create a KeyGenerator
instance. You create a KeyGenerator
instance by calling the static method getInstance()
passing as parameter the name of the encryption algorithm to create a key for. Here is an example of creating a Java KeyGenerator
instance:
Apr 12, 2016 I just ported this example to Java as that's what I now use everyday. The basic idea behind this product-key generator is that not all of the encoding/decoding logic is included in the client-installed application binary files. This design is commonly known as a Partial Key Verification System. Easy to Type In.
This example creates a KeyGenerator
instance which can generate keys for the AES encryption algorithm.
Initializing the KeyGenerator
Key Pair Generator Java Example
After creating the KeyGenerator
instance you must initialize it. Initializing a KeyGenerator
instance is done by calling its init()
method. Here is an example of initializing a KeyGenerator
instance:
The KeyGenerator
init()
method takes two parameters: The bit size of the keys to generate, and a SecureRandom
that is used during key generation.
Generating a Key
Once the Java KeyGenerator
instance is initialized you can use it to generate keys. Generating a key is done by calling the KeyGenerator
generateKey()
method. Here is an example of generating a symmetric key:
The Java KeyPairGenerator class (java.security.KeyPairGenerator
) is used to generate asymmetric encryption / decryption key pairs. An asymmetric key pair consists of two keys. The first key is typically used to encrypt data. The second key which is used to decrypt data encrypted with the first key.
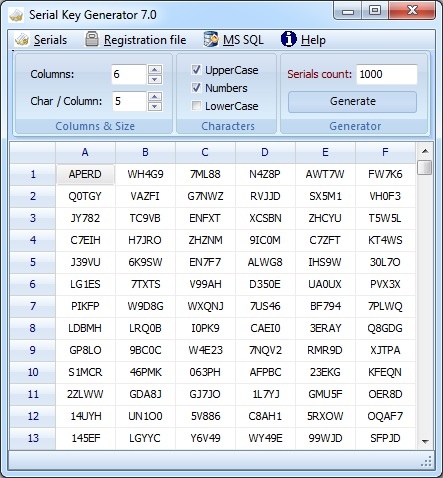
Java Code Generator
Public Key, Private Key Type Key Pairs
The most commonly known type of asymmetric key pair is the public key, private key type of key pair. The private key is used to encrypt data, and the public key can be used to decrypt the data again. Actually, you could also encrypt data using the public key and decrypt it using the private key.
The private key is normally kept secret, and the public key can be made publicly available. Thus, if Jack encrypts some data with his private key, everyone in possession of Jack's public key can decrypt it.
Creating a KeyPairGenerator Instance
To use the Java KeyPairGenerator
you must first create a KeyPairGenerator
instance. Creating a KeyPairGenerator
instance is done by calling the method getInstance()
method. Here is an example of creating a Java KeyPairGenerator
instance:
The getInstance()
method takes the name of the encryption algorithm to generate the key pair for. In this example we use the name RSA
.
Initializing the KeyPairGenerator
Random Word Generator Java
Depending on the algorithm the key pair is generated for, you may have to initialize the KeyPairGenerator
instance. Initializing the KeyPairGenerator
is done by calling its initialize()
method. Here is an example of initializing a Java KeyPairGenerator
instance:
This example initializes the KeyPairGenerator
to generate keys of 2048 bits in size.
Java Random Number Generator Code
Generating a Key Pair

To generate a KeyPair
with a KeyPairGenerator
you call the generateKeyPair()
method. Here is an example of generating a KeyPair
with the KeyPairGenerator
: